Case Study - Advanced Webhook Testing Platform for Modern Development Teams
Webhook Simulator is a sophisticated development tool that enables teams to test, debug, and validate webhook integrations in isolated environments, drastically reducing integration time and potential production issues.
- Client
- Webhook Simulator
- Year
- Service
- Web Application Development, Real-time Systems
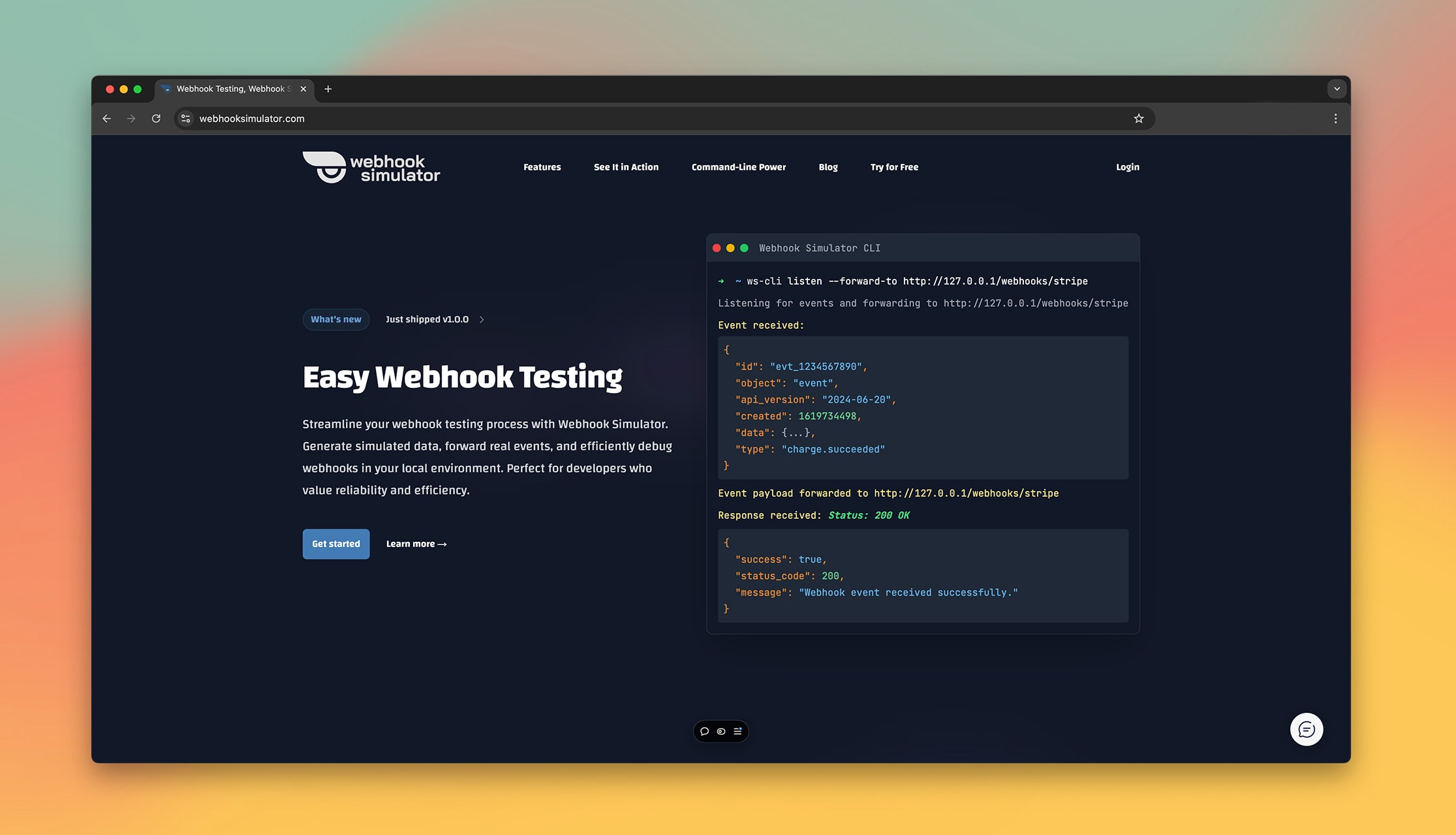
Overview
In today's interconnected software ecosystem, webhooks have become the de facto standard for real-time inter-service communication. However, testing webhook integrations has always been challenging, often requiring complex local setups or risking production environments for testing.
Webhook Simulator emerged from the need to provide developers with a robust, secure, and efficient way to test webhook integrations. Our platform allows teams to create isolated testing environments where they can simulate webhook events, inspect payloads, and debug integration issues without affecting production systems.
The application serves as a comprehensive solution for teams dealing with webhook integrations, offering features like payload validation, retry mechanisms, and detailed request inspection capabilities.
Technical Architecture
Core Components
The system is built on three main pillars:
- Web Application (Laravel Jetstream)
- Inertia.js with Vue 3 for seamless SPA experience
- Real-time updates using WebSocket connections
- SOLID principles implementation across all layers
- Comprehensive repository pattern implementation
- WebSocket Server (Go)
- High-performance real-time event broadcasting
- Efficient message queuing and delivery
- Scalable connection management
- CLI Tool (Go)
- Local webhook testing capabilities
- Integration with CI/CD pipelines
- Automated test scenario execution
What I did
- Laravel Jetstream Implementation
- Vue 3 + Inertia.js Frontend
- Websockets (Laravel Reverb)
- CLI Development
- Faster integration testing
- 90%
- Reduction in integration issues
- 75%
- More efficient error debugging
- 10x
- Supported webhook providers
- 100+
Key Features
- Isolated Testing Environments
- Separate workspace for each project
- Custom domain per environment
- Request history and logging
- Real-time Monitoring
- Live webhook inspection
- Payload validation
- Request/Response debugging
- Advanced Integration Testing
- Automated test scenarios
- Custom validation rules
- CI/CD pipeline integration
Technical Implementation
Our development approach strictly adheres to SOLID principles and modern software architecture patterns:
/**
* Repository interface for webhook events
*/
interface WebhookEventRepositoryInterface
{
/**
* Store a new webhook event
*
* @param array<string, mixed> $data
* @return WebhookEvent
*/
public function store(array $data): WebhookEvent;
/**
* Retrieve webhook events for a workspace
*
* @param string $workspaceId
* @return Collection<int, WebhookEvent>
*/
public function getWorkspaceEvents(string $workspaceId): Collection;
}
The implementation leverages Laravel's powerful features while maintaining clean architecture:
/**
* Concrete implementation of webhook event repository
*/
class WebhookEventRepository implements WebhookEventRepositoryInterface
{
/**
* @param WebhookEvent $model
*/
public function __construct(
private readonly WebhookEvent $model
) {}
/**
* {@inheritDoc}
*/
public function store(array $data): WebhookEvent
{
return $this->model->create($data);
}
}
Impact and Results
The Webhook Simulator platform has significantly improved the webhook integration process for development teams:
- Reduced integration testing time by 90%
- Eliminated the need for complex local testing setups
- Provided comprehensive debugging capabilities
- Enabled automated testing in CI/CD pipelines
Technology Stack
- Backend: Laravel 10, PHP 8.2
- Frontend: Vue 3, Inertia.js, Tailwind CSS
- Real-time: Websockets (Laravel Reverb)
- CLI: Go
- Infrastructure: Nginx, Redis, PostgreSQL